Consuming files stored in the Azure Blob Storage within Dynamics 365 Finance and Operations using X++ code
Let’s consider a scenario where third-party business application uses Azure Blob as a central repository for storing the files, which in turn needs to be consumed by Dynamics 365 finance and operations.
Following standard .Net libraries helps to consume those files:
- using Microsoft.WindowsAzure.Storage;
- using Microsoft.WindowsAzure.Storage.Blob;
- using System.IO;
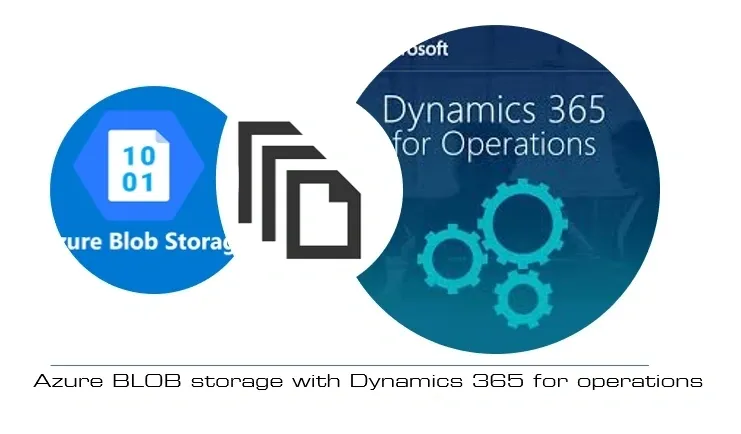
Part-2: Consuming files stored in the Azure Blob Storage within Dynamics 365 Finance and Operations using X++ code
Using the container connection created in Part-1: Azure BLOB storage with Dynamics 365 for operations – Saina Cloud Software Solutions we can perform some basic operations with blob folder, as part of this blog we cover following area:
- Get file name list
- Get file memory stream
- Read the content of the file line by line
- Read the field value from the line
) Get File Name List
This section describes how to retrieve list of file name present in the blob folder using standard classes:
- CloudBlobDirectory – Used to communicate with the blob folder
- IEnumerable – Used here to store list of file names retrieved
- IListBlobItem – Represents the list of blob items accessed
- CloudBlockBlob – Represents a blob that is uploaded as a set of blocks
Using the connection established in previous section, following code will fetch the list of files names from the cloud blob directory:
CloudBlobDirectory cloudBlobDirectory;// The directory of the blob container
container con;
cloudBlobDirectory = cloudBlobContainer.GetDirectoryReference(“SainaCloudConsulting\Test1”);//File path where the files are stored
System.Collections.IEnumerable lstEnumarable = cloudBlobDirectory.ListBlobs(false, 0, null, null);
System.Collections.IEnumerator lstEnumarator = lstEnumarable.GetEnumerator();
List filenames = new List(Types::String);
while(lstEnumarator.MoveNext())
{
IListBlobItem item = lstEnumarator.Current;
if(item is CloudBlockBlob)
{
CloudBlockBlob blob = item;
blob.FetchAttributes(null, null, null);
con = str2con(blob.name, "/");
filenames.addStart(conPeek(con,conlen(con)));
}
}
2) Get file memory stream
After retrieving the file names list, iterate the file list to get each file names, and get the file memory stream content.
- CloudBlobDirectory cloudBlobDirectory = _blobContainer.GetDirectoryReference(“SainaCloudConsulting\Test1”);
- CloudBlockBlob blob = cloudBlobDirectory.GetBlockBlobReference(“TestFile.txt”);
- System.IO.Stream memory = blob.OpenRead(null,null,null);
3) Read the content of the file line by line
Using the .Net standard IO class libraries, contents of the file can be read.
- System.IO.StreamReader streamReader = new System.IO.StreamReader(memory);
- Str strRecord = streamReader.ReadLine(); //read each line in the file
4) Read the field value from the line
Assuming the data is separated using comma field delimiter, we store each field data into container variable as shown below:
- while(!System.String::IsNullOrEmpty(strRecord))
- {
- try
- {
- Container conRecord = str2con_RU(strRecord, ‘,’);// Here the file delimiter is ‘,’.
- conPeek(conRecord, 1);// read first field data in the file line
- conPeek(conRecord, 2);// read second field data
- }
- }
This blog has covered the list of operations used to read the files from the blob container and perform necessary business action in Dynamics 365 Finance and Operation.