How to create API Services and test it in POSTMAN
Introduction:
API services refer to the set of methods, protocols, and tools provided by D365FO that allow external applications or systems to interact with the data and functionality within D365FO. These services facilitate integrations with other applications, enabling external systems (such as Power Apps, websites, mobile apps, or third-party systems) to communicate with D365FO securely and efficiently.
Scenario:
Creation of sales line through API.
High level resolution steps
We can follow the below steps to Create API Services and test it in POSTMAN.
Create a request contract class to add required input parameters.
Create a response contract class to add required output parameters.
Create a service class to add business logic.
Create a service and add the service method.
Create a service group and add the service.
Create the service URL and test it in POSTMAN.
Detailed resolution steps
Step 1: Create a request contract class and add the required parameters, here I have added the following parameters.
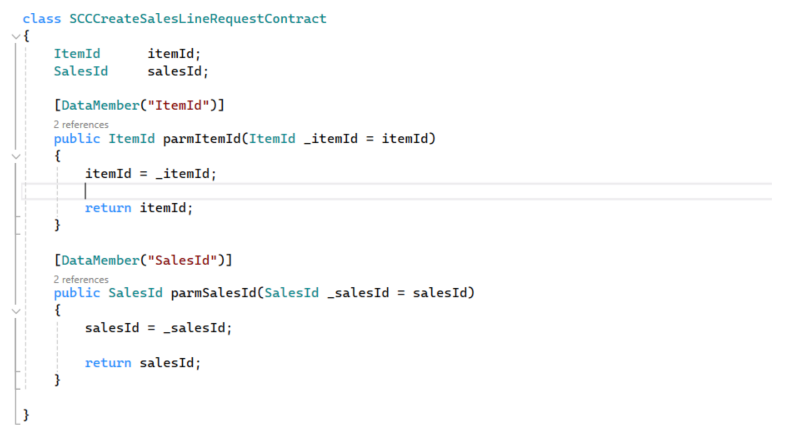
class SCCCreateSalesLineRequestContract
{
ItemId itemId;
SalesId salesId;
[DataMember("ItemId")]
public ItemId parmItemId(ItemId _itemId = itemId)
{
itemId = _itemId;
return itemId;
}
[DataMember("SalesId")]
public SalesId parmSalesId(SalesId _salesId = salesId)
{
salesId = _salesId;
return salesId;
}
}
Step 2: Create a response contract class and add the required parameters, here I have added the following parameters
class SCCCreateSalesLineResponseContract
{
RecId recId;
[DataMember("RecId")]
public RecId parmRecId(RecId _recId = recId)
{
recId = _recId;
return recId;
}
}
Step 3: Create a service class and write business logic. In this Example code has been written for creation of sales line from service by passing the contract as parameter.
class SCCCreateSalesLineServiceClass
{
public SCCCreateSalesLineResponseContract createSalesLine(SCCCreateSalesLineRequestContract _contract)
{
SalesLine salesLine;
InventDim inventDim;
SCCCreateSalesLineResponseContract sccCreateSalesLineResponseContract;
ttsbegin;
salesLine.SalesId = _contract.parmSalesId();
salesLine.ItemId = _contract.parmItemId();
salesLine.SalesQty = 1;
salesLine.createLine(NoYes::Yes, NoYes::Yes, NoYes::Yes, NoYes::Yes, NoYes::Yes, NoYes::Yes);
ttscommit;
sccCreateSalesLineResponseContract.parmRecId(salesLine.RecId);
return sccCreateSalesLineResponseContract;
}
}
Step 4: Create a service
Navigate to add new item -> Services -> Service
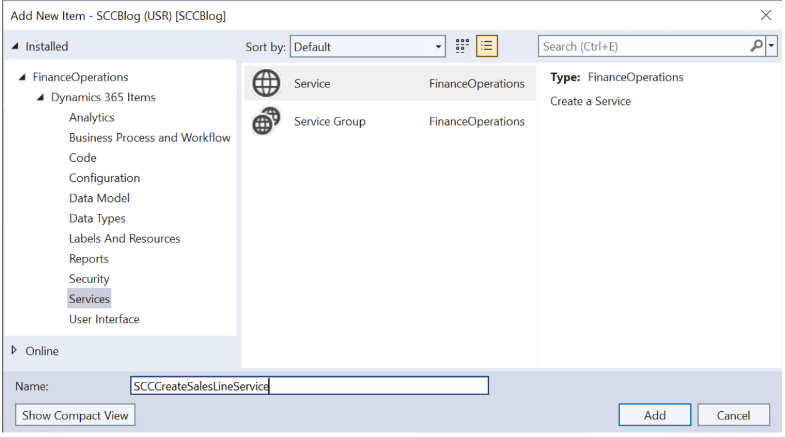
Step 5: In Service, set the class property as service class name, provide Description and External Name.
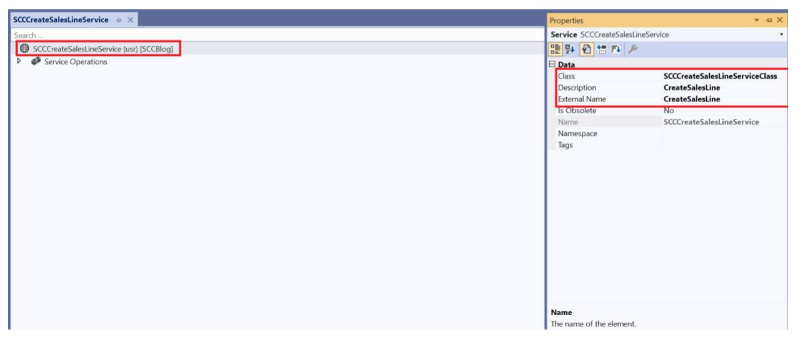
Step 6: Create a new service operation and set the property as method name.
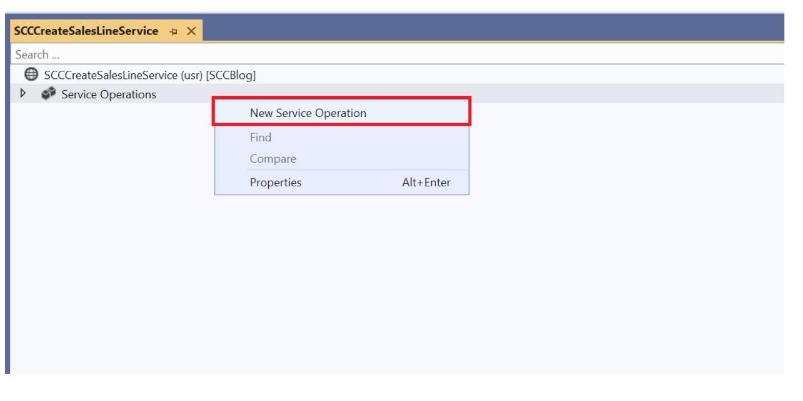
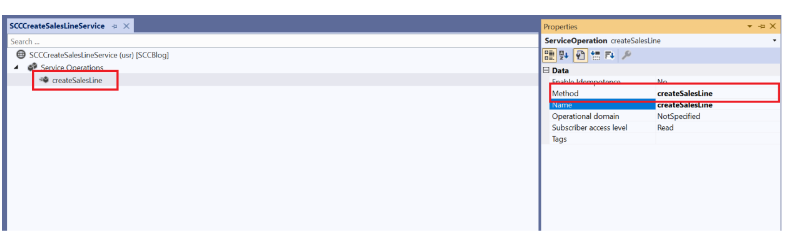
Step 7: Create a service group and add the service. Right click on service group -> New service. Set the property as service name.
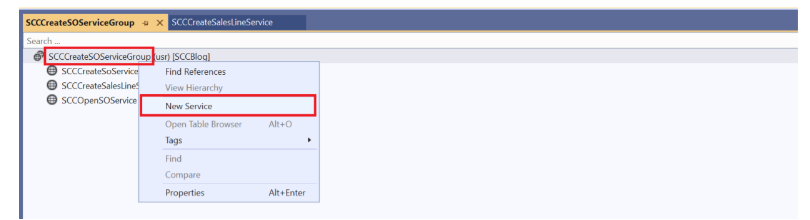
OUTPUT
First, we must generate the URL as below
Machine URL/api/services/service group name/ service name/ method
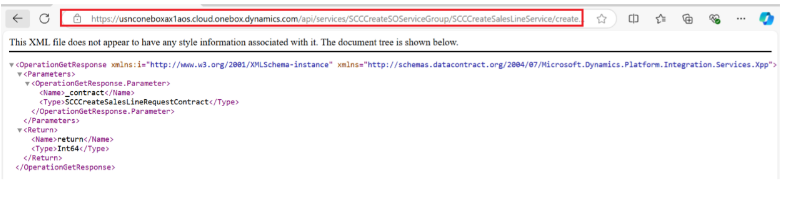
Now copy the URL. Create a new request in POSTMAN and add the URL

Now we must add the input based on parameters
Here “_contract” is the request contract class declaration in service class and give attribute names of request contract parameter. In this example, request contract parameters are ItemId and SalesId.
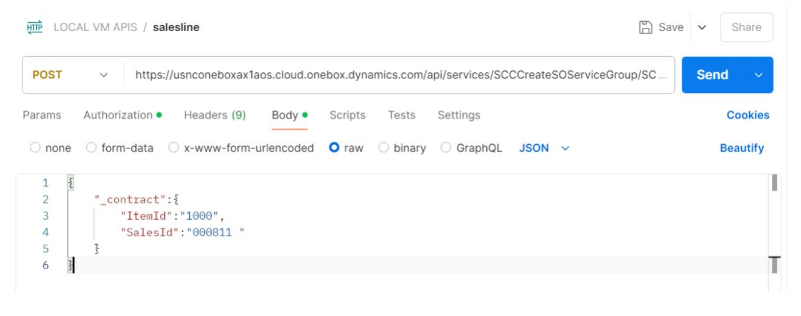
In the authorization select “Bearer Token” and add the token
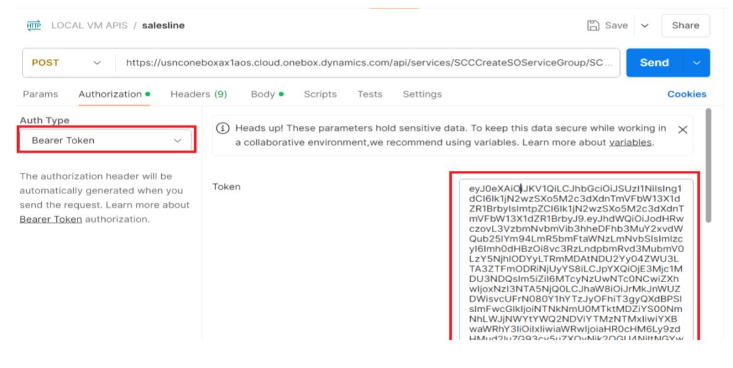
Now click on send. Response will be generated based on response contract
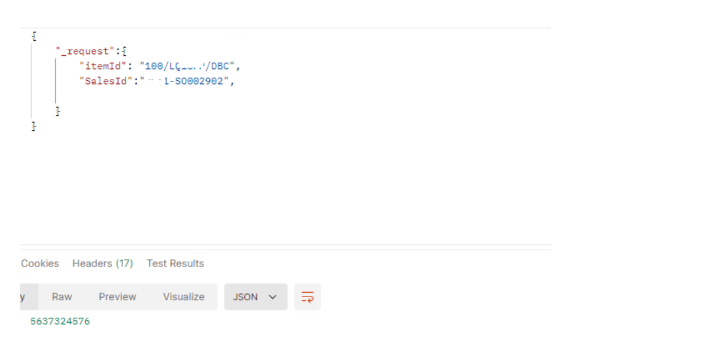