How to filter multiple values in query.
Scenario:
In some scenarios, we may need to filter multiple records dynamically, where the criteria are not predefined or fixed at the time of writing the query. This requires constructing the query in a way that can handle varying conditions efficiently.
High level resolution steps
We can follow the below steps to filter multiple values in query.
Create a runnable class and add the new query
Create a container and add the values.
Add the values in the range.
Detailed resolution steps
Step 1: Add the below code in required class
In this runnable class, I am writing code to filter out the records that match the values in the container. The container holds the values to be excluded from the table, and the remaining records to be printed
internal final class SCCTestQuery
{
///
/// Class entry point. The system will call this method when a designated menu
/// is selected or when execution starts and this class is set as the startup class.
///
/// The specified arguments.
@Override
public static void main(Args _args)
{
Query query;
QueryBuildDataSource qbds1, qbds2;
QueryRun queryRun;
SCCTestTable sccTestTable;
QueryBuildRange qbr;
container excludedValues;
query = new Query();
excludedValues = conIns(excludedValues, 1, '00006', '00001');
qbds1 = query.addDataSource(tableNum(SCCTestTable));
qbds1.addRange(fieldNum(SCCTestTable, JournalId)).value(queryNotValueCon_W(excludedValues));
queryRun = new QueryRun(query);
while(queryRun.next())
{
SCCTestTable sccTestTable1 = queryRun.get(tableNum(SCCTestTable));
info(strFmt("%1", sccTestTable1.JournalId));
}
}
}
Code Explanation:
In the following line, we can loop through all the values and store them in a container. For this example, I have hardcoded two values.
container excludedValues;query = new Query(); excludedValues = conIns(excludedValues, 1, '00006', '00001'); |
Here I am adding the range to the query
In this scenario, I am adding a range to the query by utilizing the queryNotValueCon_W function in the query range. I have used this function to pass the container buffer, which was created earlier, as the range value.
qbds1 = query.addDataSource(tableNum(SCCTestTable)); qbds1.addRange(fieldNum(SCCTestTable,JournalId)).value(queryNotValueCon_W(excludedValues)); |
OUTPUT
In my custom table currently, I have the following records
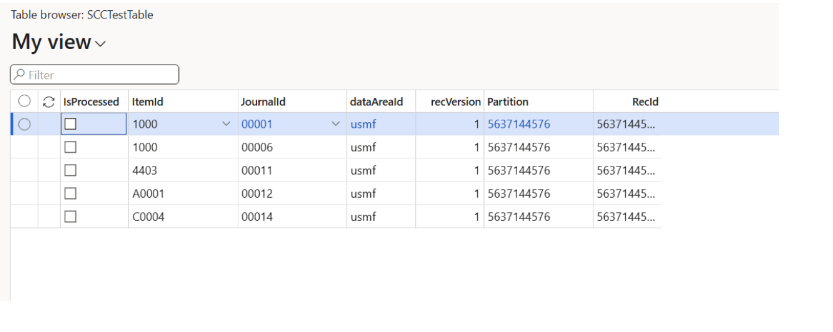
When I run the runnable class, the hardcoded values are successfully excluded, and the remaining records are displayed.
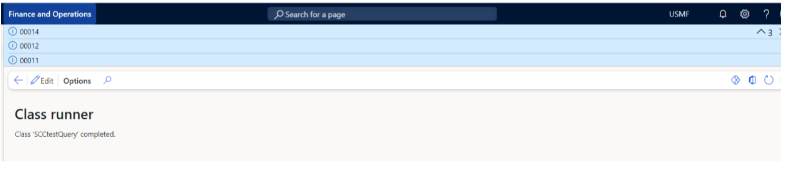