public class POLMobileServiceTable extends common
{
///
/// When i initialize a record, this method will be triggered
/// This method is not responsible for saving the record to database
/// Rather it assigns value to BUFFER
///
public void initValue()
{
super();
this.ServiceStartDate = systemDateGet();
this.ExpectedDeliveryDate = systemDateGet() + 5;
}
///
/// Validate delivery date and service amount
///
///
public boolean validateWrite()
{
boolean ret;
ret = super();
//If all the previous valdiations have passed?
if (ret)
{
//Then check for the date validation
if (this.ServiceStartDate > this.ExpectedDeliveryDate)
{
//error("Expected delivery date cannot be lesser than service start date.");
//ret = false;
ret = checkFailed("Expected delivery date cannot be lesser than service start date.");
}
//If all the previous valdiations have passed?
if (ret && this.ExpectedServiceAmount <= 0)
{
ret = checkFailed("Expected service amount should be specified.");
}
}
return ret;
}
///
///
///
///
public boolean validateDelete()
{
boolean ret;
ret = super();
if (ret)
{
if (this.ServiceStatus == POLServiceStatus::Delivered)
{
ret = checkFailed("Service status with Delivered, cannot be allowed to delete.");
}
}
return ret;
}
///
///
///
///
public void modifiedField(FieldId _fieldId)
{
super(_fieldId);
switch(_fieldId)
{
case fieldNum(POLMobileServiceTable, ModelID):
//1st way
//if (this.ModelID == "Samsung")
//{
// this.ExpectedServiceAmount = 2000;
//}
//else if (this.ModelID == "Sony")
//{
// this.ExpectedServiceAmount = 1500;
//}
//else if (this.ModelID == "Apple")
//{
// this.ExpectedServiceAmount = 3000;
//}
//2nd way
//POLModelTable polModelTable;
//select * from polModelTable
// where polModelTable.ModelID == this.ModelID; //current selection
//3rd way
//POLModelTable polModelTable = POLModelTable::find(this.ModelID);
//this.ExpectedServiceAmount = polModelTable.MinimumServiceAmount;
//best way for writing
this.ExpectedServiceAmount = POLModelTable::find(this.ModelID).MinimumServiceAmount;
break;
}
}
///
///
///
public static POLMobileServiceTable find(POSServiceID _serviceID, boolean _forUpdate = false)
{
POLMobileServiceTable polMobileServiceTable;
if (_serviceID)
{
polMobileServiceTable.selectForUpdate(_forUpdate);
select * from polMobileServiceTable
index hint ServiceIdx
where polMobileServiceTable.ServiceID == _serviceID;
}
return polMobileServiceTable;
}
}
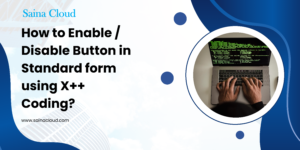