How to purge any files from Azure blob storage container using X++?
Scenario: User needs to access the storage container files to delete them from D365 F&O. Here, in this scenario I am explaining step by step how we can delete the container files using X++ code.
High Resolution steps
- Create a runnable class.
- Write the business logic to delete the blob container files in main method.
- In Azure blob storage, manually stored the files in the container.
- Delete the files using runnable class and test container whether the files are deleted.
Detailed Resolution steps
Step 1: Create the runnable class (NAGConnectionClass) which is used to test the containers files are getting deleted from azure storage container.
Step2: Write the below logic to delete the blob container files in main method.
Code Snippet:
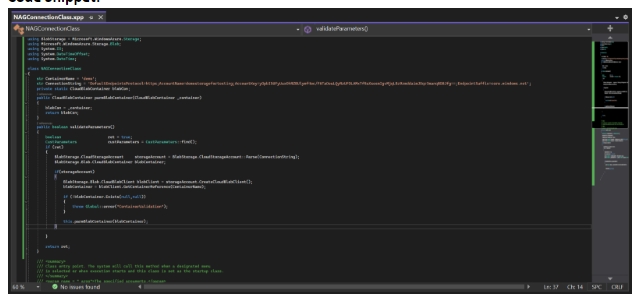
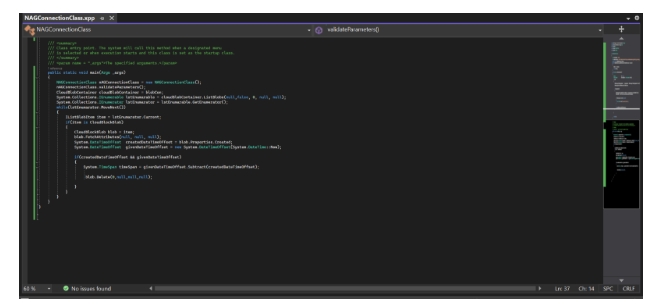
Code:
Copy button for small sizeusing BlobStorage = Microsoft.WindowsAzure.Storage;
using Microsoft.WindowsAzure.Storage.Blob;
using System.IO;
using System.DateTimeOffset;
using System.DateTime;
class NAGConnectionClass
{
str ContainerName = 'demo';
str ConnectionString = 'DefaultEndpointsProtocol=https;AccountName=demostoragefortesting;AccountKey=yOpbI5UFyUuxOhRZBUlymf4oc/F6TaOeuLQyRzUFOLAMxTf9aXsesmIgvMjqLBz9zmddaimJXqr3manq8O8Jfg==;EndpointSuffix=core.windows.net';
private static CloudBlobContainer blobCon;
public CloudBlobContainer parmBlobContainer(CloudBlobContainer _container)
{
blobCon = _container;
return blobCon;
}
public boolean validateParameters()
{
boolean ret = true;
if (ret)
{
BlobStorage.CloudStorageAccount storageAccount = BlobStorage.CloudStorageAccount::Parse(ConnectionString);
BlobStorage.Blob.CloudBlobContainer blobContainer;
if(storageAccount)
{
BlobStorage.Blob.CloudBlobClient blobClient = storageAccount.CreateCloudBlobClient();
blobContainer = blobClient.GetContainerReference(ContainerName);
if (!blobContainer.Exists(null,null))
{
throw Global::error("ContainerValidation");
}
this.parmBlobContainer(blobContainer);
}
}
return ret;
}
public static void main(Args _args)
{
CloudBlobContainer cloudBlobContainer = blobCon;
System.Collections.IEnumerable lstEnumarable = cloudBlobContainer.ListBlobs(null,false, 0, null, null);
System.Collections.IEnumerator lstEnumarator = lstEnumarable.GetEnumerator();
while(lstEnumarator.MoveNext())
{
IListBlobItem item = lstEnumarator.Current;
if(item is CloudBlockBlob)
{
CloudBlockBlob blob = item;
blob.FetchAttributes(null, null, null);
System.DateTimeOffset createdDateTimeOffset = blob.Properties.Created;
System.DateTimeOffset givenDateTimeOffset = new System.DateTimeOffset(System.DateTime::Now);
if(createdDateTimeOffset && givenDateTimeOffset)
{
System.TimeSpan timeSpan = givenDateTimeOffset.Subtract(createdDateTimeOffset);
blob.Delete(0,null,null,null);
}
}
}
}
}
Code Explanation:
We have manually provided the container name and connection string. Using the validate parameters method, we are verifying the container name and connection string where the files are stored. In the main method, we establish the connection between the Azure Blob Storage container and dynamics 365 finance and operations then delete the files from the container.
Output
Files are manually stored in the container, as shown in the below screenshot.
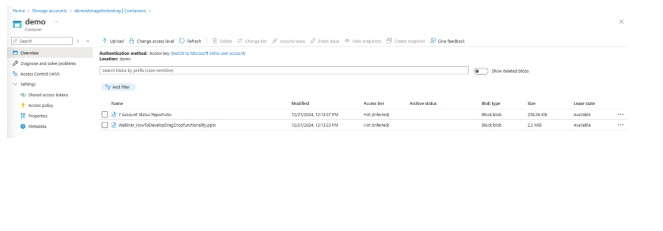
To perform the runnable class,it will purge the files in the container.
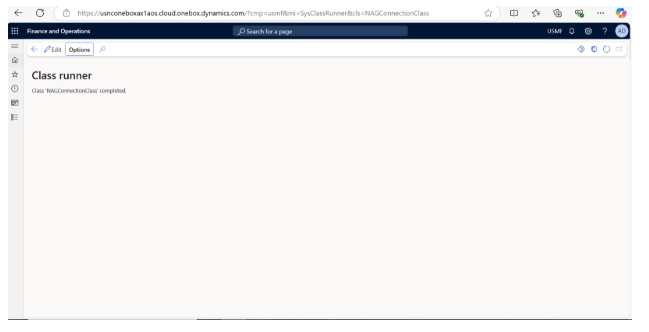
Once the Runnable class is completed, it will be refreshed in the container, and the files will be purged.
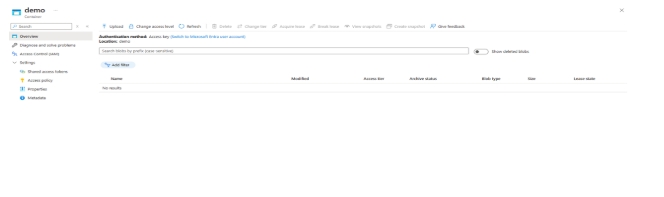