How to send mail using template in X++.
Scenario: Whenever an error occurs or the code process fails, an automated email should be sent to the management team notifying them of the issue.
High level resolution steps
- Create the mail template from D365 UI.
- Provide the mail body to the template.
- Create runnable class to write the business logic.
- Retrieve mail template and send the mail.
- Ensure the sent mail to recipient .
Detailed resolution steps
Code Snippet:
internal final class SCCEmailNotification
{
///
/// Class entry point. The system will call this method when a designated menu
/// is selected or when execution starts and this class is set as the startup class.
///
/// The specified arguments.
public static void main(Args _args)
{
SCCEmailNotification::generateEmail("error occured while running sainacloud batch");
}
public static void generateEmail(str _message)
{
str emailToAddress = 'dheerajg@sainacloud.com';
Map templateTokens;
str emailSenderAddr, emailSubject, emailBody;
SysEmailTable emailTable = SysEmailTable::find('ErrorMsg');
if(emailTable.RecId)
{
SysEmailMessageTable messageTable = SysEmailMessageTable::find(emailTable.EmailId, emailTable.DefaultLanguage);
if(messageTable.Subject && messageTable.Mail && emailTable.SenderAddr)
{
SysMailerMessageBuilder messageBuilder = new SysMailerMessageBuilder();
messageBuilder.addTo(emailToAddress);
messageBuilder.setSubject(messageTable.Subject + ‘ ’ + _message);
messageBuilder.setBody(messageTable.Mail);
messageBuilder.setFrom(emailTable.SenderAddr);
SysMailerFactory::getNonInteractiveMailer().sendNonInteractive(messageBuilder.getMessage());
}
}
}
}
Step1: Create the “Error message” mail template in the organization administration module.
Path: Organization administration à setup à organization email template
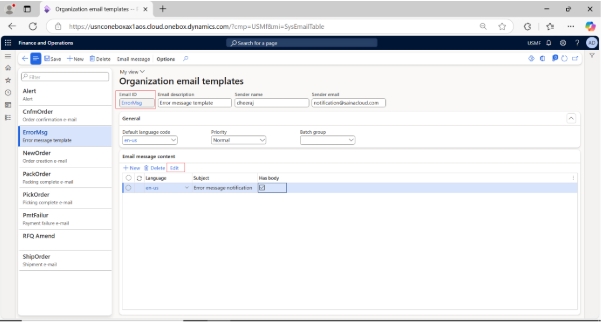
Step 2: Once the template is created, click on the edit button to upload the HTML mail template. HTML content will be displayed in the below box.
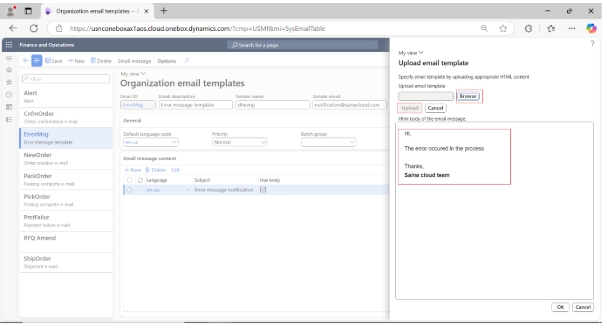
Step 3: I have created the runnable class to send the mail using template which configured in the Organization Administration module. In generateEmail() method hardcoded the email recipients mail address this can be parameterized. Using the template id we are getting the email template information.
public static void generateEmail(str _message)
{
str emailToAddress = 'dheerajg@sainacloud.com';
Map templateTokens;
str emailSenderAddr, emailSubject, emailBody;
SysEmailTable emailTable = SysEmailTable::find('ErrorMsg');
Step 4: Checking whether template is present in the SysEmailTable, if exist. Fetching the template details such as subject, mail body, and sender address from the SysEmailMessageTable. Using it constructing the mail and sending mail using the SysMailFactory class with non-interactive manner that means will not get any confirmation window before sending the mail.
if(emailTable.RecId)
{
SysEmailMessageTable messageTable = SysEmailMessageTable::find(emailTable.EmailId, emailTable.DefaultLanguage);
if(messageTable.Subject && messageTable.Mail && emailTable.SenderAddr)
{
SysMailerMessageBuilder messageBuilder = new SysMailerMessageBuilder();
messageBuilder.addTo(emailToAddress);
messageBuilder.setSubject(messageTable.Subject + ‘ ’ + _message);
messageBuilder.setBody(messageTable.Mail);
messageBuilder.setFrom(emailTable.SenderAddr);
SysMailerFactory::getNonInteractiveMailer().sendNonInteractive(messageBuilder.getMessage());
}
}
Output
Navigate to the UI. Run the batch job using the URL. Change the batch name according to yours. Below is the reference URL to run the batch job (runnable batch job).
Once the batch job is completed, it sends the mail to the provided recipient mail address in the batch job class.
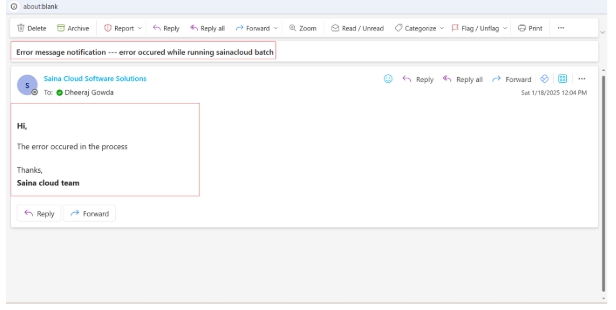