- To update a corresponding field with additional text when a new contact record is created.
- When we provide First name and last name and save the record we should get “Hello World” and followed by the first and last name that is input in fields.
High level resolution steps
- C# Code plugin in Visual Studio Community 2022
- Use Plugin Registration tool
- Use Dynamics CRM as front end and login with your Microsoft credentials
Detailed resolution steps
Step 1:- Write a C# code in VS Community Edition 2022 as shown below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Microsoft.Xrm.Sdk; //Install this tools in Nuget Package manager
using System.ServiceModel; //Install this service model tool in Nuget Package
namespace MyPlugins
{
public class HelloWorld : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
// Extract the tracing service for use in debugging sandboxed plug-ins.
// If you are not registering the plug-in in the sandbox, then you do
// not have to add any tracing service related code.
ITracingService tracingService =
(ITracingService)serviceProvider.GetService(typeof(ITracingService));
// Obtain the execution context from the service provider.
IPluginExecutionContext context = (IPluginExecutionContext)
serviceProvider.GetService(typeof(IPluginExecutionContext));
// Obtain the organization service reference which you will need for
// web service calls.
IOrganizationServiceFactory serviceFactory =
(IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
// The InputParameters collection contains all the data passed in the message request.
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
// Obtain the target entity from the input parameters.
Entity entity = (Entity)context.InputParameters["Target"];
try
{
// Plug-in business logic goes here.
string firstName = string.Empty;
//Read form attribute values
if (entity.Attributes.Contains("firstname"))
{
firstName = entity.Attributes["firstname"].ToString();
}
string lastName =
entity.Attributes["lastname"].ToString();
//assign data to attributes
entity.Attributes.Add("description", "Hello World" + " " + firstName + " " + lastName);
}
catch (FaultException ex)
{
throw new InvalidPluginExecutionException("An error occurred in MyPlug-in.", ex);
}
catch (Exception ex)
{
tracingService.Trace("MyPlugin: {0}", ex.ToString());
throw;
}
}
}
}
}
Step 2:-
Save the code and build the solution and then sign the assembly in the following steps:
Hit Alt+Enter in keyboard >> Click on “Signing” on the left pane >> and sign the assembly.
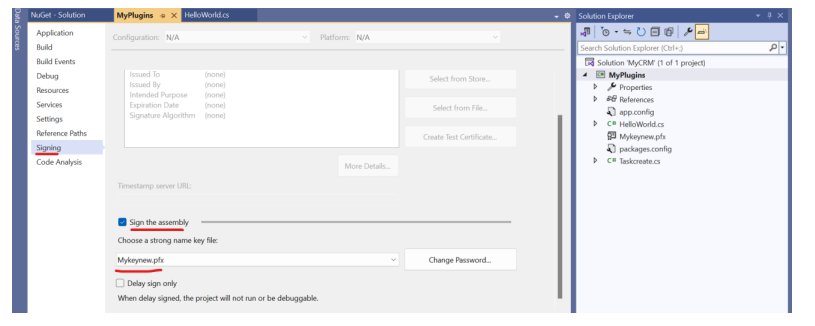
Step 3:-
Install Plugin registration tool in the following path as shown in the below picture:
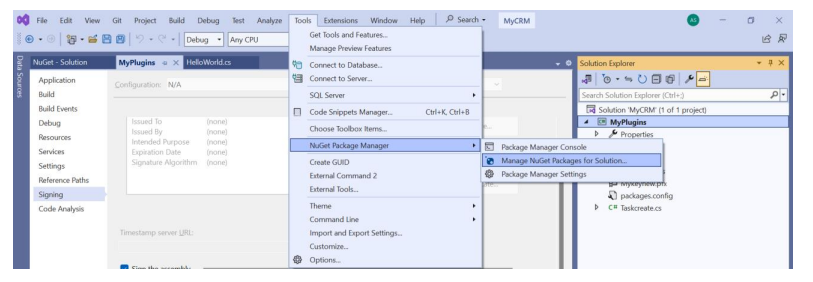
Then, search for Plugin Registration in the search box and install the package for your project as shown in the below picture:
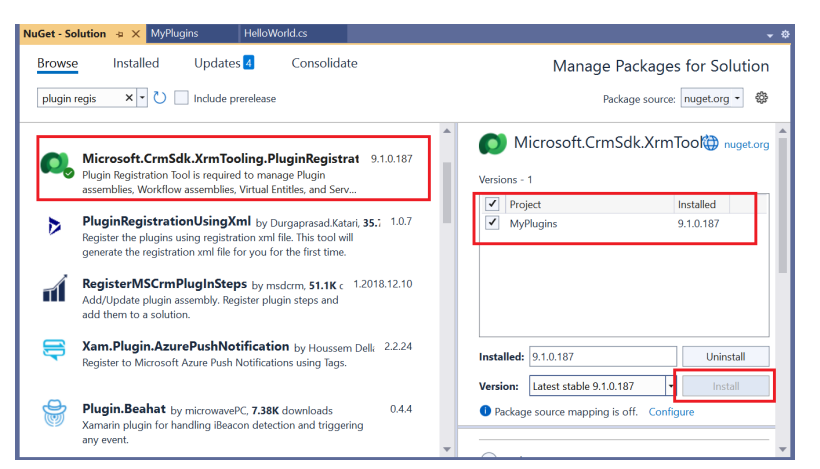
Step 4:-
Once package is installed open the “Plugin Registration tool” that is installed in your desktop and login with your Microsoft credentials that is used to login to your CRM as well.
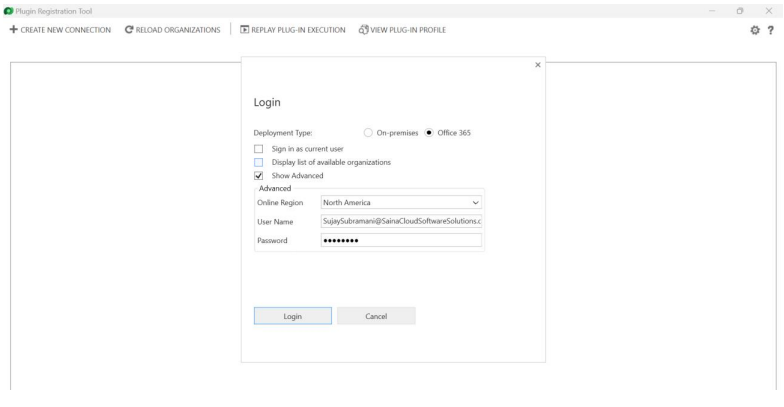
Next, register new assembly after logging in as shown below:
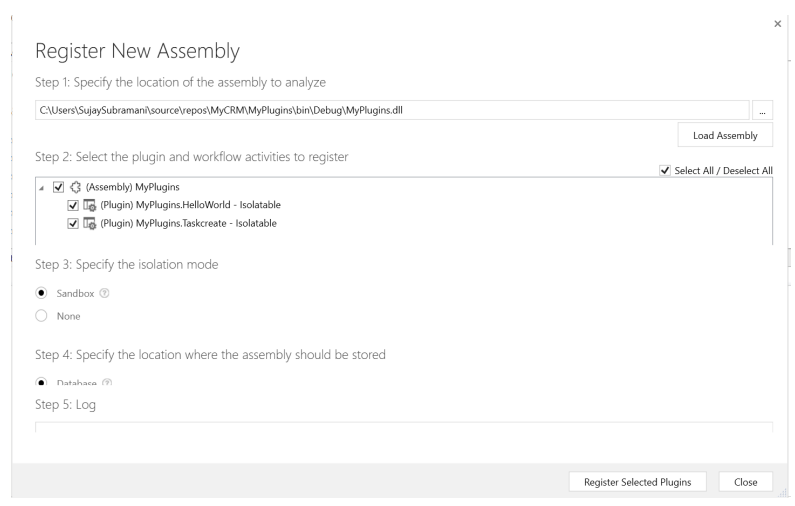
Step 5:–
Register new step under the assembly you created by right clicking on the assembly.
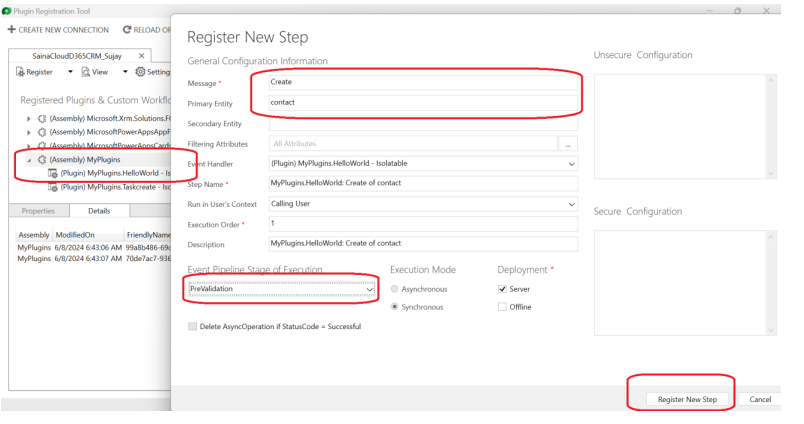
In Message field – Give Create as the option (because in our scenario, on creation of contact record only the operation should happen)
Primary Entity – Give the entity name from your CRM Dataverse (Contact entity in our case)
Stage of Execution – Give Pre-Validation (This means your plugin will run before the form is validated)
Output:
Once I Input First name and last name as “Peter” and “John”
The description field will be updated as “Hello World John and Peter” once we create a record and save it.
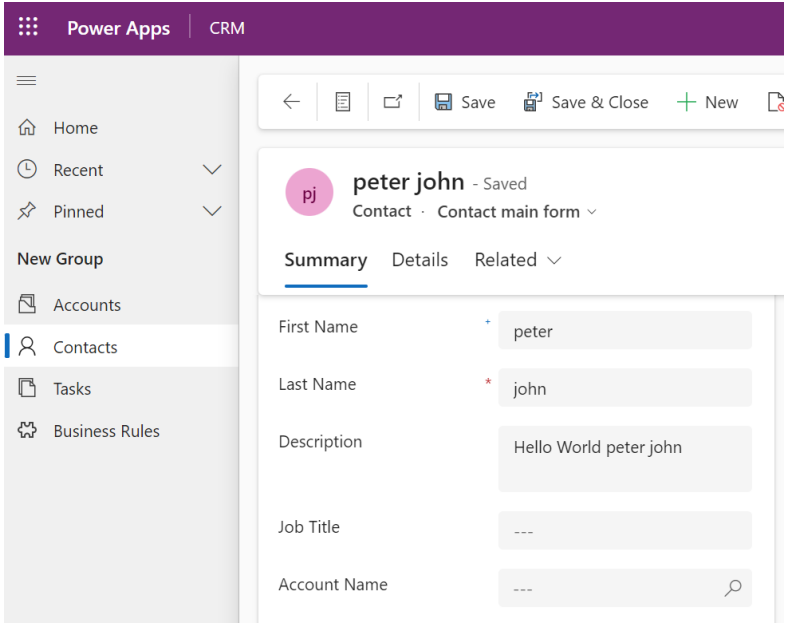
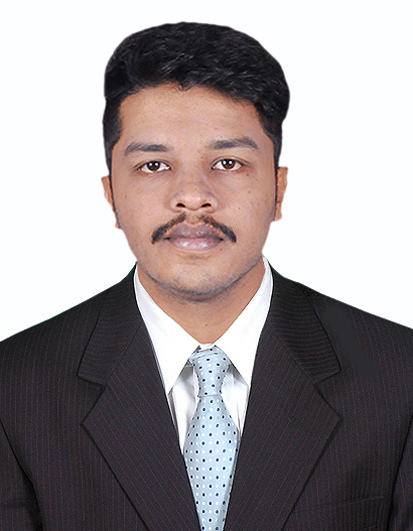
Technical Consultant – Enjoys created low code applications using Power platform, skilled at creating automate flow using Power automate and Power Virtual agents.