Automate The Export Of DIXF Projects Using X++ Code:
The DMF project should automatically export to Excel when the user runs the batch job, ensuring data is saved without manual intervention.
High level resolution steps
- Create a simple batch job.
- Implement the logic in a service class.
- Run the batch job from the user interface.
Step 1: Create a simple batch job; Refer the below link to create it.
Video blog: Sys Operation framework batch job with parameter X++ – Saina Cloud Software Solutions
Step 2: Implement the business logic in a service class. This code ensures the DMF project is exported and made available for download automatically when the batch job runs.
class SCCSysOperationDemoService extends SysOperationServiceBase
{
public void processDemo()
{
DMFDefinitionGroupName dmGroupName = 'SCC employee project export';
str downloadUrl;
DMFDefinitionGroupEntity dmfDefinitionGroupEntity;
str exportFileName;
try
{
select firstonly dmfDefinitionGroupEntity
where dmfDefinitionGroupEntity.DefinitionGroup == dmGroupName;
DMFExecutionId executionId = DMFUtil::setupRunExecution(dmGroupName);
DataImportFramework::MoveToStaging(executionId);
SharedServiceSubmitFileID exportedFileId = DMPackageExporter::exportToFileV2(dmGroupName, executionId, dmfDefinitionGroupEntity.Entity, dmfDefinitionGroupEntity.Source);
if (exportedFileId != '')
{
downloadUrl = DMFDataPopulation::getAzureBlobReadUrl(str2Guid(exportedFileId));
System.Uri uri = new System.Uri(downloadUrl);
str fileExt;
if (uri != null)
{
fileExt = System.IO.Path::GetExtension(uri.LocalPath);
Filename filename = strFmt('Export - %1', fileExt);
System.IO.Stream stream = File::UseFileFromURL(downloadUrl);
File::SendFileToUser(stream, filename);
}
}
}
catch
{
error('DMF export failed');
}
}
}
Code Explanation:
- Variable Declaration:
- dmfGroupName: The name of the DMF project group to be exported.
- downloadUrl: The URL to download the exported file.
- dmfDefinitionGroupEntity: Entity representing the DMF group.
- exportFileName: Name of the export file.
- Try-Catch Block:
- Select DMF Group Entity: Fetches the first entity from DMFDefinitionGroupEntity that matches dmfGroupName.
- Setup Execution: Initializes a new DMF execution using DMFUtil::setupNewExecution.
- Move to Staging: Moves data to the staging environment using DataImportFramework::MoveToStaging.
- Export to File: Exports the DMF group to a file using DMFPackageExporter::exportToFileV2.
- Download URL: If the export is successful, gets the Azure Blob storage URL for the exported file using DMFDataPopulation::getAzureBlobReadUrl.
- Extract File Extension: Extracts the file extension from the URL.
- Send File to User: Creates a filename and sends the file to the user using File::UseFileFromURL and File::SendFileToUser.
- Error Handling:
- If any errors occur during the export process, an error message “DMF export failed” is displayed
public void processDemo()
{
DMFDefinitionGroupName dmfGroupName = 'SCC employee project export';
str downloadUrl;
DMFDefinitionGroupEntity dmfDefinitionGroupEntity;
str exportFileName;
try
{
select firstonly dmfDefinitionGroupEntity
where dmfDefinitionGroupEntity.DefinitionGroup == dmfGroupName;
DMFExecutionId executionId = DMFUtil::setupNewExecution(dmfGroupName);
DataImportFramework::MoveToStaging(executionId);
SharedServiceUnitFileID exportedFileId = DMFPackageExporter::exportToFileV2(dmfGroupName, executionId, dmfDefinitionGroupEntity.Entity, dmfDefinitionGroupEntity.Source);
if (exportedFileId != '')
{
downloadUrl = DMFDataPopulation::getAzureBlobReadUrl(str2Guid(exportedFileId));
System.Uri uri = new System.Uri(downloadUrl);
str fileExt;
if (uri != null)
{
fileExt = System.IO.Path::GetExtension(uri.LocalPath);
}
Filename filename = strFmt('Export - %1', fileExt);
System.IO.Stream stream = File::UseFileFromURL(downloadUrl);
File::SendFileToUser(stream, filename);
}
}
catch
{
error('DMF export failed');
}
}
Output:
When user can run the batch job in UI. The excel file downloads in the local folder.
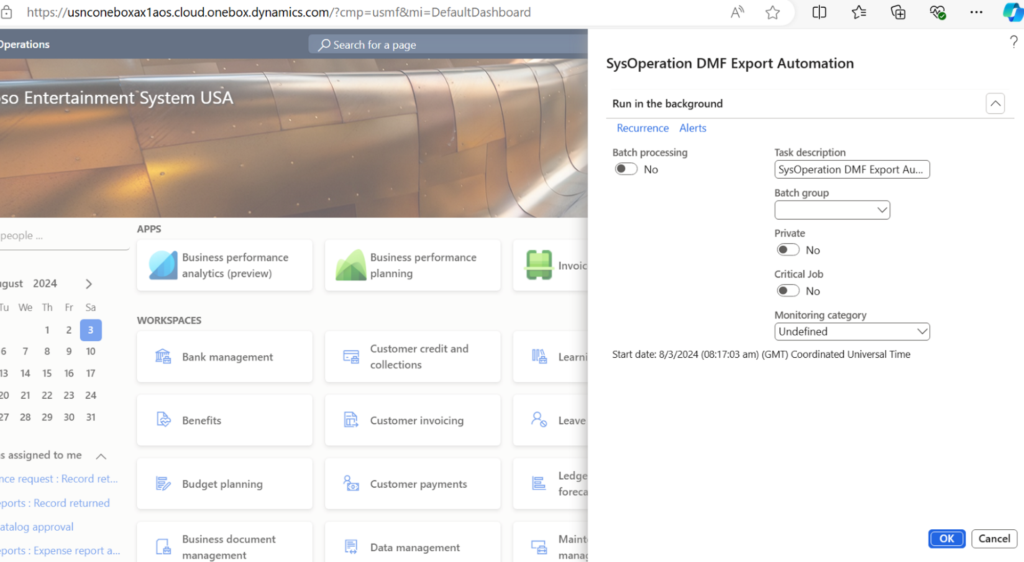
Exported files can be available in the local folder.
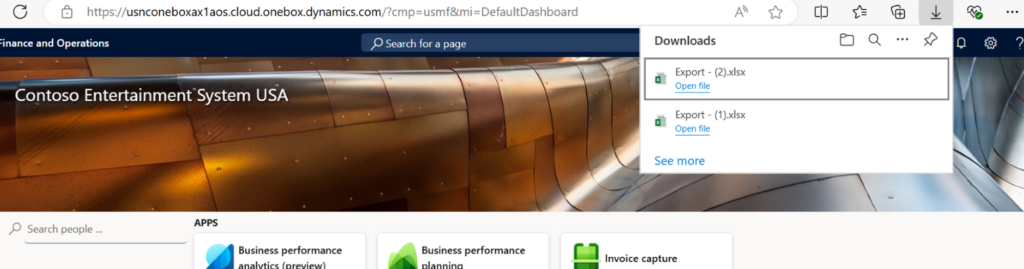
User can be able to open the downloaded file.
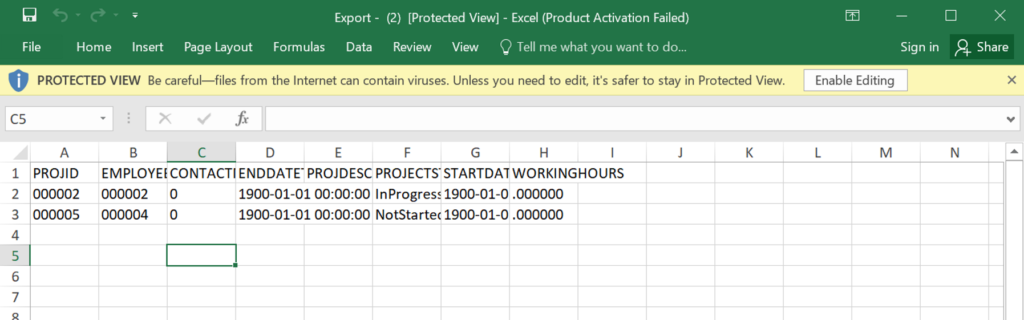
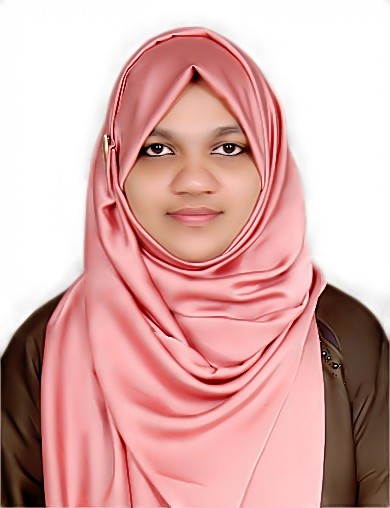
Ayisha Sidhika M I
Dynamics 365 for Operations Technical Consultant | Passionate about coding, customizations, and workflow optimization